So, there are a lot of confusion in developers mind regarding Dependency Inversion Principle, Dependency Injection and Inversion of Control, that’s why I tried to explain as par my experience. We need to understand a bit of Software Design Principle & Software Design Pattern. Dependency Inversion Principle (DIP) is a Software design principle and Inversion of Control (IoC) is a Software design pattern. Let's see what is Software design principle and pattern.
Software Design Principle:
Principle provides us only guideline to achieve some task. Principle tells us what is right and what is wrong. It doesn’t say us how to solve problem. It just gives some guideline so that we can design good software and avoid bad design. Some principles are DRY, OCP, DIP etc.
Software Design Pattern:
Pattern is a general reusable solution to a commonly occurring problem within a given context in software design. Some patterns are factory pattern, Decorator pattern etc.
What is Dependency Inversion Principle
-
High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
The Dependency Inversion principle (DIP) helps to decouple your code by ensuring that you depend on abstractions rather than concrete implementations.
Let’s, try to understand this principle. Following figure shows high level depends on low level interface. So causing any changes in high level class need to think about all the interfaces. Similarly, when new low level class comes then again high level class need to change which makes complexity on maintenance and it violates open close principle.
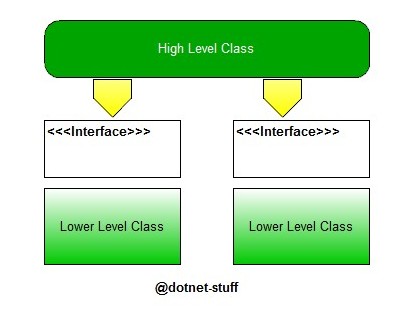
Now let’s, try to apply Dependency Inversion Principle. Following figure shows higher level class defines the interface and higher level class doesn’t depend on lower level class directly instead depend on interface. And, lower level classes implements interface defined by higher level class so it is not required to make changes in higher level class to change when new implementation arrived.
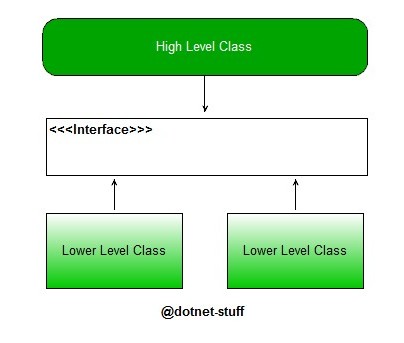
What is Inversion of Control (Ioc)
• Inversion of Control (IoC) is a Software design pattern. IoC is a way that provide abstraction. A way to change the control. IoC gives some ways to implement DIP. If we want to make independent higher level module from the lower level module then we have to invert the control so that low level module not controlling interface and creation of the object. Finally IoC gives a way to invert the control. We can divide Ioc into three ways:
Interface Inversion:
Let’s invert interfaces. Let's try to understand by considering an example, Here we have no benefit because every time we have to change our provider class and service consumer class had to maintain all the changes. Now we have a method called interface inversion. Let invert the interfaces.
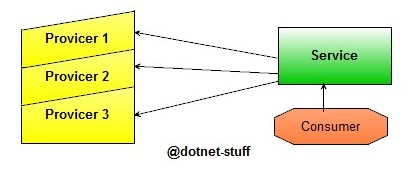
We remove all the interfaces from lower level classes and make single interface defined by Provider class. What we did here just invert the interface. These changes allow us to achieve a lot of benefit. We don’t need to change neither Provider class nor Service class. Following figure shows it.
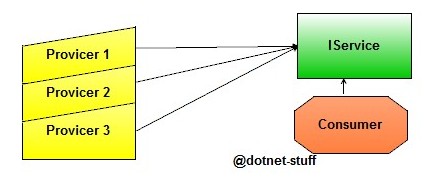
Flow Inversion:
Invert the flow of control. Let's consider a simple example. We are writing a program to get some information from a user and we using a command line enquiry. We might do it something like this
Console.WriteLine("What is your name?");
string strName = Console.ReadLine();
Console.WriteLine("What is your age?");
string strAge = Console.ReadLine();
Console.WriteLine("Out put is: " + ProcessData(strName, strAge));
private string ProcessData(string userName,string age)
{
string strData = string.Empty;
// TODO: some logic here
return strData;
}
In above code is in control: it decides when to ask questions, when to read responses, and when to process those results.
However if We want to do this in a window application, We would do it by designing a window and writing some code as shown below.
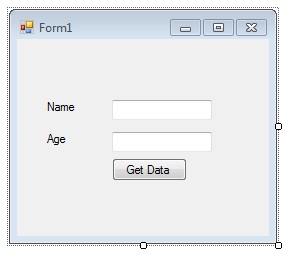
private void button1_Click(object sender, EventArgs e)
{
ProcessData(textBox1.Text, textBox2.Text);
}
private string ProcessData(string userName, string age)
{
string strData = string.Empty;
// TODO: some logic here
return strData;
}
Now, There's a big difference now in the flow of control between these programs - in particular the control of when the ProcessData methods are called. In the command line we control when the method are called, but in the window example we don't. Instead we hand control over to the window system by making an event for the button and button decides when to call my method. This phenomenon is Inversion of Control (also known as the Hollywood Principle - "Don't call us, we'll call you").
Creation Inversion:
Invert the creation of control. Factory Pattern is one of the best examples of Creation Inversion. We had already discussed it as a separate article.
A typical diagram shows how Dependency Inversion Principle, Inversion of Control and Dependency Injection can be organized.
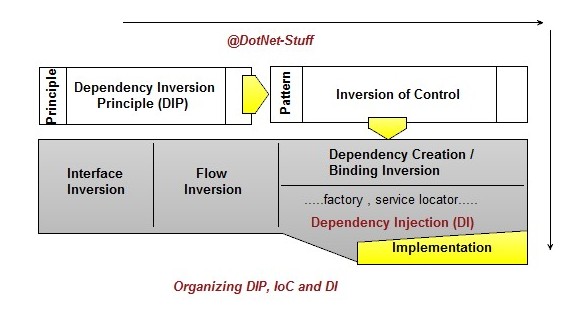
This is the view how all things can be organized together. There are other possible ways to implement Dependency. At the top is DIP which is a way of designing software. It doesn’t say how to make independent module. IoC provides the way of applying DPI principle. But still IoC doesn’t provide us specific implementation. It gives some methods so that we can invert the control. If we want to invert control using Binding inversion or dependency creation then we can achieve it by implementing dependency injection (DI).
We have discussed Dependency Injection (DI) as a separate article; here we only try to know the core concept of Dependency Injection (DI). A type of IoC where we move the creation and binding of dependency outside of the class that depends on it. In normal object are created inside of the dependent class and bounded inside the dependent class. In Dependency Injection (DI) it is done from outside of the dependent class. There are three type of Dependency Injection (DI).
-
Constructor Injection
- Setter Injection
- Interface Injection
Summary:
Here I was trying to explain about Dependency Inversion Principle, Dependency Injection and Inversion of Control (DIP, IoC and DI). You can find some more articles about Ioc Container and Dependency injection in this blog. I had written the articles in details.
Reference:
http://www.codeproject.com/Articles/538536/A-curry-of-Dependency-Inversion-Principle-DIP-Inve#IoC and DIP